React JS Payments SDK
This page gives you an overview of Decentro's React JS based payments SDK.
This documentation provides detailed insights into leveraging our SDK for Android, iOS, and React applications.
Note: This document assumes that you have a foundational understanding of React and have established a project beforehand. For those who are new to React, we suggest referring to the Getting Started guide before proceeding.
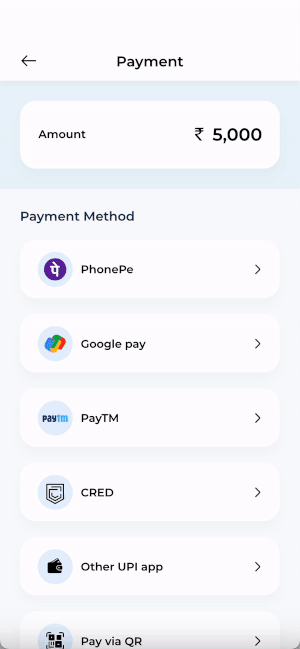
Key features
- Offers a consistent user experience, with low-level details handled by the SDK.
- Supports the latest UPI Deep links APIs.
- Scalable and performant architecture for efficient processing of payments.
- Offers accurate polling statuses on time to reduce the transaction TAT.
- Easy integration with React JS applications.
- Custom QR code based payments for ease of access of making the payments.
- Validate any VPA before initiating the transaction in the checkout flow.
- Initiate a collection request to any valid UPI handle to collect the payment.
- Easy to consume without in depth knowledge.
- No need to implement HTTP client every time.
- Prevent mistakes like validation of required field and only known methods/content
- Combine multiple calls in single line of code.
Getting Started
Installation
Install using below command
npm i @decentro1/collections-payment-sdk-web
yarn add @decentro1/collections-payment-sdk-web
Registry setup
echo \"@decentro1:registry\" \"https://gitlab.com/api/v4/packages/npm/\" >> .yarnrc
.npmrc setup
@decentro1:registry=https://gitlab.com/api/v4/projects/<project-id>/packages/npm/
//gitlab.com/api/v4/projects/<project-id>/packages/npm/:_authToken=<auth-token>
To get the project-id and auth-token please contact support [email protected].
Implementation
The npm package installed in the previous step contains all the components to be used by the client.
(Note: Parameters that are optional while using APIs (Check here) are Mandatory during SDK consumption)
Custom flow
For businesses that prefer to have their own custom checkout flows, they can simply consume the collections SDKs and start collecting the payment.
In this API, the customer or the platform generates UPI link with required amount that can be paid through any of the PSP apps on the customer's mobile through a generic UPI payment link or UPI deep links for PSP apps or QR scan.
Sample Usage
import {
useLinkWithoutUI
} from "@decentro1/collections-payment-sdk-web";
function App() {
const urlHeader = {
client_id: "String", //<your_client_id>
client_secret: "String", // <your_client_secret>
module_secret: "String", // <your_module_secret>
provider_secret: "String", // <YOUR_PROVIDER_SECRET>
};
// API body
const urlBody = {
reference_id: "String",
payee_account: "Int", //payee account number
amount: "Int", // amount to be deducted
purpose_message: "String", // payment message
generate_qr: "Int", // 0 = do not generate QR , 1 = to generate QR
expiry_time: "Int", //expiry time for payment
customized_qr_with_logo: "Int",
generate_uri: "Int", // to generate the deep links
};
// Using a query hook automatically fetches data and returns query values
let {
isLoading,
error,
upiPaymentOptionData,
} = useLinkWithoutUI({
urlHeaderData: urlHeader,
urlBodyData: urlBody,
});
return (
<div className="App">
<button onClick={refetchPaymentStatus} >
hit api again
</button>
</div>
);
}
export default App;
Query Hook Return Values
Param | Description |
---|---|
isLoading / isLoadingPaymentStatus | When true, indicates that the query is currently loading for the first time, and has no data yet. This will be true for the first request fired off, but not for subsequent requests. |
error | The error result if present while getting multiple payments links query. |
upiPaymentOptionData | The latest returned result for multiple payments links query. |
This API can be used to get the status of the collection triggered using the Payment link/Collection request.
Sample Usage
import {
useGTStatus
} from "@decentro1/collections-payment-sdk-web";
function App() {
const urlHeader = {
client_id: "String", //<your_client_id>
client_secret: "String", // <your_client_secret>
module_secret: "String", // <your_module_secret>
provider_secret: "String", // <YOUR_PROVIDER_SECRET>
};
// Using a query hook automatically fetches data and returns query values
let { data, loading, error, refetchPaymentStatus } = useGTStatus({
urlHeaderData: urlHeader,
decentroTxnID: "String",
polling: false, // if true = the polling will start at defined interval , false = no polling will return result once
});
return (
<div className="App">
<button onClick={refetchPaymentStatus} >
hit api again
</button>
</div>
);
}
export default App;
Query hook return values
1st Column | 2nd Column |
---|---|
decentroTxnId | Decentro transfer ID used to get the status of the collection triggered using the Payment link/Collection query. |
data | The latest returned result for the status of the collection triggered using the Payment link/Collection query. |
Loading | The latest returned result for the status of the collection triggered using the Payment link/Collection query. |
error | The error result if present while getting the status of the collection triggered using the Payment link/Collection query. |
refetchPaymentStatus | Function that can be used to again initiate getting the status of the collection triggered using the Payment link/Collection query. |
c) Validate VPA
Hook is used to check if a particular UPI-ID (Virtual Payment Address) is valid or not. Decentro recommends platforms use this before triggering a collection request or even doing UPI payouts.
Sample Usage
import {
useUPIvalidation
} from "@decentro1/collections-payment-sdk-web";
function App() {
const urlHeader = {
client_id: "String", //<your_client_id>
client_secret: "String", // <your_client_secret>
module_secret: "String", // <your_module_secret>
provider_secret: "String", // <YOUR_PROVIDER_SECRET>
};
let { data, loading, error, rePost } = useUPIvalidation({
urlHeaderData: urlHeader,
upi_id: "string", //upi ID that needs to be validated
});
return (
<div className="App">
<button onClick={rePost} >
hit api again
</button>
</div>
);
}
export default App;
Query Hook Return Values
Param | Description |
---|---|
Loading | When true, it indicates that the query is currently loading for the first time and has no data yet. This will be true for the first request fired off but not for subsequent requests. |
error | The error result, if present, while getting a UPI validation query. |
data | The latest returned result for getting a UPI validation query. |
rePost | A function that can be used to initiate a UPI validation query again. |
Collection requests powered by Decentro for platforms to request money to specific UPI ID.Send a payment collection request to a specific UPI ID (for example - abcd@bank).
Sample Usage
import {
useCollectionReq
} from "@decentro1/collections-payment-sdk-web";
function App() {
const urlHeader = {
client_id: "String", //<your_client_id>
client_secret: "String", // <your_client_secret>
module_secret: "String", // <your_module_secret>
provider_secret: "String", // <YOUR_PROVIDER_SECRET>
};
let { data, loading, error, rePost } = useCollectionReq({
urlHeaderData: urlHeader,
urlBodyData: {
reference_id: randomUUID(),
payer_upi: "String", // UPI ID in which collection request need to be send
payee_account: "Int",
amount: "Int",
purpose_message: "String",
expiry_time: 30,
},
});
return (
<div className="App">
<button onClick={rePost} >
hit api again
</button>
</div>
);
}
export default App;
Query Hook Return Values
Column | Description |
---|---|
Loading | When true, this indicates that the query is currently loading for the first time and has no data yet. This will be true for the first request fired off, but not for subsequent requests. |
Error | This represents the error result, if present, while sending Collection requests query. |
Data | This is the latest returned result for sending Collection requests query. |
rePost | This is a function that can be used to initiate Collection requests query again. |
In built checkout flow
For businesses that does not want to implement their own UI screen, an optional checkout flow is provided by Decentro to enhance the end customer payment experience.
Sample Usage
import { CollectionUIComponent } from "@decentro1/collections-payment-sdk-web";
function App() {
const urlHeader = {
client_id: "String", //<your_client_id>
client_secret: "String", // <your_client_secret>
module_secret: "String", // <your_module_secret>
provider_secret: "String", // <YOUR_PROVIDER_SECRET>
};
// API body
const urlBody = {
reference_id: "String",
payee_account: "Int", //payee account number
amount: "Int", // amount to be deducted
purpose_message: "String", // payment message
generate_qr: "Int", // 0 = do not generate QR , 1 = to generate QR
expiry_time: "Int", //expiry time for payment
customized_qr_with_logo: "Int",
generate_uri: "Int", // to generate the deep links
};
const [linkAPIResponse, setlinkAPIResponse] = useState(null);
const [paymentAPIResponse, setPaymentAPIResponse] = useState(null);
const [upiValidateAPIResponse, setUpiValidateAPIResponse] = useState(null);
const [collectionAPIResponse, setCollectionAPIResponse] = useState(null);
return (
<div className="App">
<CollectionUIComponent
urlHeaderData={urlHeader}
urlBodyData={urlBody}
linkGenerationAPIRes={setlinkAPIResponse} //will return api response for generate payment link
gtsStatusAPIRes={setPaymentAPIResponse} //will return api response for check payment status
upiValidateAPIRes={setUpiValidateAPIResponse} //will return api response for validate UPI ID
collectionReqAPIRes={setCollectionAPIResponse} //will return api response for issue collection request
/>
</div>
);
}
export default App;
CSS implementation
// inside index.css
@import url('https://fonts.googleapis.com/css2?family=Montserrat:ital,wght@0,100;0,200;0,300;0,400;0,500;0,600;0,800;0,900;1,700&display=swap');
*{
box-sizing: border-box;
}
Updated 7 months ago